Articulation Controller#
Overview#
Articulation controller is the low level controller that controls joint position, joint velocity, and joint effort in Isaac Sim. The articulation controller can be interfaced using Python and Omnigraph.
Note
Angular units are expressed in radians while angles in USD are expressed in degrees and will be adjusted accordingly by the articulation controller.
Python Interface#
Create the articulation controller#
There are several ways to create the articulation controller. The articulation controller is usually created implicitly by applying articulation on a robot prim through the SingleArticulation
class.
However, the articulation controller can be created directly by importing the controller class before the simulation starts, but this approach will require you to create or pass in the Articulation
during initialization.
The snippet below will load and apply articulation on a franka robot.
1import isaacsim.core.utils.stage as stage_utils 2from isaacsim.core.prims import SingleArticulation 3usd_path = "/Path/To/Robots/Franka/franka_alt_fingers.usd" 4prim_path = "/World/envs/env_0/panda" 5 6# load the Franka Panda robot USD file 7stage_utils.add_reference_to_stage(usd_path, prim_path) 8# wrap the prim as an articulation 9prim = SingleArticulation(prim_path=prim_path, name="franka_panda")
1from isaacsim.core.api.controllers.articulation_controller import ArticulationController 2articulation_controller = ArticulationController()
Initialize the controller#
After the simulation is started, the robot articulation must be initialzied before any commands can be passed to the robot.
The more common approach is by initializing the single articulation object that you have created earlier, this will initialize the articulation controller and articulation view stored in the SingleArticulation object
1prim.initialize()
After the simulation starts, the articulation controller must be initialzied with an articulation view. Articulation view is the backend for selecting the joints and applying joint actions.
For example, the code snippet below assumes you have a Franka robot in the scene at
/World/envs/env_0/panda
, and you have created aarticulation_view
for the Franka robot already separately.1articulation_controller.initialize(articulation_view)
Articulation Action#
Joint controls commands are packaged in ArticulationAction
objects first, before sending them to the articulation controller. The articulation controller allows you to specify the command joint postion, velocity and effort, as well as joint indicies of the joints actuated.
If the joint indice is empty, the articulation action will assume the command will apply to all joints of the robot, and if any of the command is 0, articulation action will assume it is unactuated.
For example, the snippet below creates the command that closes the franka robot fingers: panda_finger_joint1 (7) and panda_finger_joint2 (8) to 0.0
1import numpy as np
2from isaacsim.core.utils.types import ArticulationAction
3
4action = ArticulationAction(joint_positions=np.array([0.0, 0.0]), joint_indices=np.array([7, 8]))
This snippet creates the command that moves all the robot joints to the indicated position
1import numpy as np
2from isaacsim.core.utils.types import ArticulationAction
3
4action = ArticulationAction(joint_positions=np.array([0.0, -1.0, 0.0, -2.2, 0.0, 2.4, 0.8, 0.04, 0.04]))
Important
Make sure the joint commands matches the order and the number of joint indices passed in to the articulation action. If joint indice is not passed in, make sure the command matches the number of joints in the robot.
Note
A joint can only be controlled by one control method. For example a joint cannot be controlled by both desired position and desired torque
Apply Action#
The apply_action
funciton in both SingleArticulation
and ArticulationController
classes will apply the ArticulationAction
you created earlier to the robot.
1prim.apply_action(action)
1articulation_controller.apply_action(action)
Omnigraph Interface#
The articulation controller can also be accessed via a omnigraph node. In this approach, the robot prim information
Input#
Input Commands |
description |
---|---|
execIn |
Input execution |
targetPrim |
The prim with the robot articulation root, leave this empty if robotPath is populated |
robotPath |
Path to the robot articulation root, leave this empty if targetPrim is populated |
jointIndices |
indices of the commanded joints, leave this empty if all joints are controlled or if jointNames is populated |
jointNames |
names of the commanded joints, leave this empty if all joints are controlled or if jointIndices is populated |
positionCommand |
desired position command, leave this empty if the robot is not controlled by target joint positions |
velocityCommand |
desired velocity command, leave this empty if the robot is not controlled by target joint velocity |
effortCommand |
effort command, leave this empty if the robot is not controlled by effort |
Important
Make sure the joint commands matches the order and the number of joint indices or joint names passed in to the articulation action node. If joint indice or joint names are not passed in, make sure the command matches the number of joints in the robot.
Note
A joint can only be controlled by one control method. For example a joint cannot be controlled by both desired position and desired torque
See mock_robot_rigged
for sample usage, this asset can be found in the Asset Browser at Samples > Rigging > MockRobot > mock_robot_rigged.usd
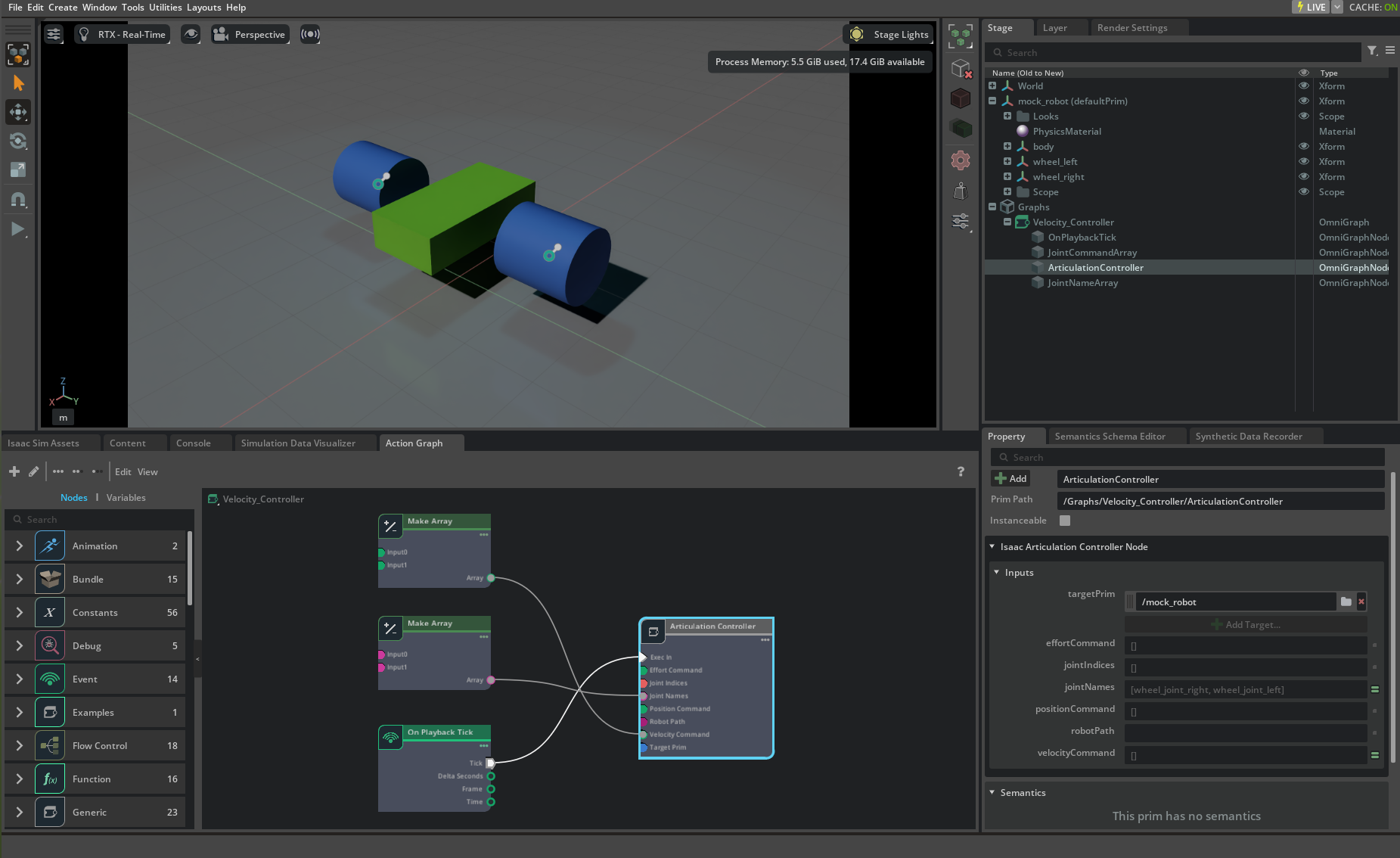