[isaacsim.sensors.rtx] Isaac Sim Isaac Sensor Simulation#
Version: 13.6.3
Provides APIs for RTX-based sensors, including RTX Lidar & RTX Radar.
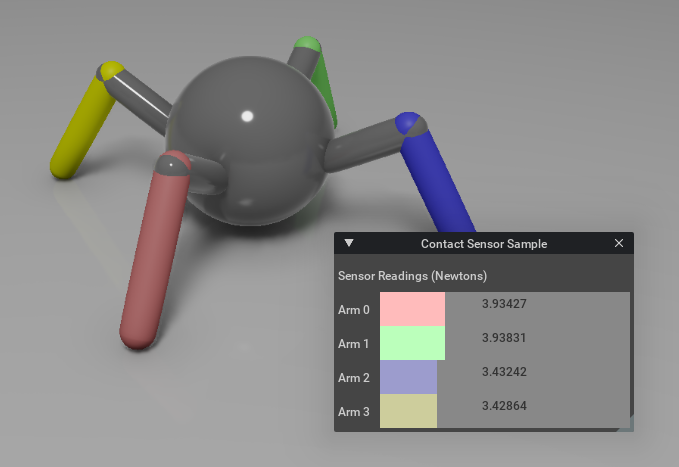
Enable Extension#
The extension can be enabled (if not already) in one of the following ways:
Define the next entry as an application argument from a terminal.
APP_SCRIPT.(sh|bat) --enable isaacsim.sensors.rtx
Define the next entry under [dependencies]
in an experience (.kit
) file or an extension configuration (extension.toml
) file.
[dependencies]
"isaacsim.sensors.rtx" = {}
Open the Window > Extensions menu in a running application instance and search for isaacsim.sensors.rtx
.
Then, toggle the enable control button if it is not already active.
API#
Python API#
Commands
Sensors
Commands#
- class IsaacSensorCreateRtxLidar(*args: Any, **kwargs: Any)#
Bases:
Command
- class IsaacSensorCreateRtxIDS(*args: Any, **kwargs: Any)#
Bases:
Command
- class IsaacSensorCreateRtxRadar(*args: Any, **kwargs: Any)#
Bases:
Command
Sensors#
- class LidarRtx(
- prim_path: str,
- name: str = 'lidar_rtx',
- position: ndarray | None = None,
- translation: ndarray | None = None,
- orientation: ndarray | None = None,
- config_file_name: str | None = None,
- firing_frequency: int | None = None,
- firing_dt: float | None = None,
- rotation_frequency: int | None = None,
- rotation_dt: float | None = None,
- valid_range: Tuple[float, float] | None = None,
- scan_type: str | None = None,
- elevation_range: Tuple[float, float] | None = None,
- range_resolution: float | None = None,
- range_accuracy: float | None = None,
- avg_power: float | None = None,
- wave_length: float | None = None,
- pulse_time: float | None = None,
Bases:
BaseSensor
- get_render_product_path() str #
- Returns:
gets the path to the render product used by the lidar
- Return type:
string
- get_current_frame() dict #
- initialize(physics_sim_view=None) None #
Create a physics simulation view if not passed and using PhysX tensor API
Note
If the prim has been added to the world scene (e.g.,
world.scene.add(prim)
), it will be automatically initialized when the world is reset (e.g.,world.reset()
).- Parameters:
physics_sim_view (omni.physics.tensors.SimulationView, optional) – current physics simulation view. Defaults to None.
Example:
>>> prim.initialize()
- post_reset() None #
Reset the prim to its default state (position and orientation).
Note
For an articulation, in addition to configuring the root prim’s default position and spatial orientation (defined via the
set_default_state
method), the joint’s positions, velocities, and efforts (defined via theset_joints_default_state
method) are imposedExample:
>>> prim.post_reset()
- resume() None #
- pause() None #
- is_paused() bool #
- add_point_cloud_data_to_frame()#
- remove_point_cloud_data_from_frame()#
- add_linear_depth_data_to_frame()#
- remove_linear_depth_data_from_frame()#
- add_intensities_data_to_frame()#
- remove_intensities_data_from_frame()#
- add_azimuth_range_to_frame()#
- remove_azimuth_range_from_frame()#
- add_horizontal_resolution_to_frame()#
- remove_horizontal_resolution_from_frame()#
- add_range_data_to_frame()#
- remove_range_data_from_frame()#
- add_azimuth_data_to_frame()#
- remove_azimuth_data_from_frame()#
- add_elevation_data_to_frame()#
- remove_elevation_data_from_frame()#
- get_horizontal_resolution() float #
- get_horizontal_fov() float #
- get_num_rows() int #
- get_num_cols() int #
- get_rotation_frequency() float #
- get_depth_range() Tuple[float, float] #
- get_azimuth_range() Tuple[float, float] #
- enable_visualization()#
- disable_visualization()#
- apply_visual_material(
- visual_material: VisualMaterial,
- weaker_than_descendants: bool = False,
Apply visual material to the held prim and optionally its descendants.
- Parameters:
visual_material (VisualMaterial) – visual material to be applied to the held prim. Currently supports PreviewSurface, OmniPBR and OmniGlass.
weaker_than_descendants (bool, optional) – True if the material shouldn’t override the descendants materials, otherwise False. Defaults to False.
Example:
>>> from isaacsim.core.api.materials import OmniGlass >>> >>> # create a dark-red glass visual material >>> material = OmniGlass( ... prim_path="/World/material/glass", # path to the material prim to create ... ior=1.25, ... depth=0.001, ... thin_walled=False, ... color=np.array([0.5, 0.0, 0.0]) ... ) >>> prim.apply_visual_material(material)
- get_applied_visual_material() VisualMaterial #
Return the current applied visual material in case it was applied using apply_visual_material or it’s one of the following materials that was already applied before: PreviewSurface, OmniPBR and OmniGlass.
- Returns:
the current applied visual material if its type is currently supported.
- Return type:
Example:
>>> # given a visual material applied >>> prim.get_applied_visual_material() <isaacsim.core.api.materials.omni_glass.OmniGlass object at 0x7f36263106a0>
- get_default_state() XFormPrimState #
Get the default prim states (spatial position and orientation).
- Returns:
an object that contains the default state of the prim (position and orientation)
- Return type:
Example:
>>> state = prim.get_default_state() >>> state <isaacsim.core.utils.types.XFormPrimState object at 0x7f33addda650> >>> >>> state.position [-4.5299529e-08 -1.8347054e-09 -2.8610229e-08] >>> state.orientation [1. 0. 0. 0.]
- get_local_pose() Tuple[ndarray, ndarray] #
Get prim’s pose with respect to the local frame (the prim’s parent frame)
- Returns:
first index is the position in the local frame (with shape (3, )). Second index is quaternion orientation (with shape (4, )) in the local frame
- Return type:
Tuple[np.ndarray, np.ndarray]
Example:
>>> # if the prim is in position (1.0, 0.5, 0.0) with respect to the world frame >>> position, orientation = prim.get_local_pose() >>> position [0. 0. 0.] >>> orientation [0. 0. 0.]
- get_local_scale() ndarray #
Get prim’s scale with respect to the local frame (the parent’s frame)
- Returns:
scale applied to the prim’s dimensions in the local frame. shape is (3, ).
- Return type:
np.ndarray
Example:
>>> prim.get_local_scale() [1. 1. 1.]
- get_visibility() bool #
- Returns:
true if the prim is visible in stage. false otherwise.
- Return type:
bool
Example:
>>> # get the visible state of an visible prim on the stage >>> prim.get_visibility() True
- get_world_pose() Tuple[ndarray, ndarray] #
Get prim’s pose with respect to the world’s frame
- Returns:
first index is the position in the world frame (with shape (3, )). Second index is quaternion orientation (with shape (4, )) in the world frame
- Return type:
Tuple[np.ndarray, np.ndarray]
Example:
>>> # if the prim is in position (1.0, 0.5, 0.0) with respect to the world frame >>> position, orientation = prim.get_world_pose() >>> position [1. 0.5 0. ] >>> orientation [1. 0. 0. 0.]
- get_world_scale() ndarray #
Get prim’s scale with respect to the world’s frame
- Returns:
scale applied to the prim’s dimensions in the world frame. shape is (3, ).
- Return type:
np.ndarray
Example:
>>> prim.get_world_scale() [1. 1. 1.]
- is_valid() bool #
Check if the prim path has a valid USD Prim at it
- Returns:
True is the current prim path corresponds to a valid prim in stage. False otherwise.
- Return type:
bool
Example:
>>> # given an existing and valid prim >>> prims.is_valid() True
- is_visual_material_applied() bool #
Check if there is a visual material applied
- Returns:
True if there is a visual material applied. False otherwise.
- Return type:
bool
Example:
>>> # given a visual material applied >>> prim.is_visual_material_applied() True
- property name: str | None#
Returns: str: name given to the prim when instantiating it. Otherwise None.
- property non_root_articulation_link: bool#
Used to query if the prim is a non root articulation link
- Returns:
True if the prim itself is a non root link
- Return type:
bool
Example:
>>> # for a wrapped articulation (where the root prim has the Physics Articulation Root property applied) >>> prim.non_root_articulation_link False
- property prim: pxr.Usd.Prim#
Returns: Usd.Prim: USD Prim object that this object holds.
- property prim_path: str#
Returns: str: prim path in the stage
- set_default_state(
- position: Sequence[float] | None = None,
- orientation: Sequence[float] | None = None,
Set the default state of the prim (position and orientation), that will be used after each reset.
- Parameters:
position (Optional[Sequence[float]], optional) – position in the world frame of the prim. shape is (3, ). Defaults to None, which means left unchanged.
orientation (Optional[Sequence[float]], optional) – quaternion orientation in the world frame of the prim. quaternion is scalar-first (w, x, y, z). shape is (4, ). Defaults to None, which means left unchanged.
Example:
>>> # configure default state >>> prim.set_default_state(position=np.array([1.0, 0.5, 0.0]), orientation=np.array([1, 0, 0, 0])) >>> >>> # set default states during post-reset >>> prim.post_reset()
- set_local_pose(
- translation: Sequence[float] | None = None,
- orientation: Sequence[float] | None = None,
Set prim’s pose with respect to the local frame (the prim’s parent frame).
Warning
This method will change (teleport) the prim pose immediately to the indicated value
- Parameters:
translation (Optional[Sequence[float]], optional) – translation in the local frame of the prim (with respect to its parent prim). shape is (3, ). Defaults to None, which means left unchanged.
orientation (Optional[Sequence[float]], optional) – quaternion orientation in the local frame of the prim. quaternion is scalar-first (w, x, y, z). shape is (4, ). Defaults to None, which means left unchanged.
Hint
This method belongs to the methods used to set the prim state
Example:
>>> prim.set_local_pose(translation=np.array([1.0, 0.5, 0.0]), orientation=np.array([1., 0., 0., 0.]))
- set_local_scale(
- scale: Sequence[float] | None,
Set prim’s scale with respect to the local frame (the prim’s parent frame).
- Parameters:
scale (Optional[Sequence[float]]) – scale to be applied to the prim’s dimensions. shape is (3, ). Defaults to None, which means left unchanged.
Example:
>>> # scale prim 10 times smaller >>> prim.set_local_scale(np.array([0.1, 0.1, 0.1]))
- set_visibility(visible: bool) None #
Set the visibility of the prim in stage
- Parameters:
visible (bool) – flag to set the visibility of the usd prim in stage.
Example:
>>> # make prim not visible in the stage >>> prim.set_visibility(visible=False)
- set_world_pose(
- position: Sequence[float] | None = None,
- orientation: Sequence[float] | None = None,
Ses prim’s pose with respect to the world’s frame
Warning
This method will change (teleport) the prim pose immediately to the indicated value
- Parameters:
position (Optional[Sequence[float]], optional) – position in the world frame of the prim. shape is (3, ). Defaults to None, which means left unchanged.
orientation (Optional[Sequence[float]], optional) – quaternion orientation in the world frame of the prim. quaternion is scalar-first (w, x, y, z). shape is (4, ). Defaults to None, which means left unchanged.
Hint
This method belongs to the methods used to set the prim state
Example:
>>> prim.set_world_pose(position=np.array([1.0, 0.5, 0.0]), orientation=np.array([1., 0., 0., 0.]))
Omnigraph Nodes#
The extension exposes the following Omnigraph nodes:
Settings#
Other Settings#
The extension changes some settings of the application or other extensions, which are listed in the table below.
Application/extension setting |
Value |
---|---|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|